REST API Salesforce to Salesforce Integration
- India Cloud
- May 11, 2022
- 3 min read
Updated: May 24, 2022
What you will learn from this:
1) Rest API Apex - encapsulate your data, expose desired data to external system
2) Http Callouts Apex - reach out to Salesforce to fetch exposed data
3) How to use Postman with Salesforce
4) Use Connected apps
5) How to work with Salesforce OAuth2, Customer key, Customer secret
6) Remote Site settings
What you will need:
Two Salesforce Developer Orgs, one is Server Org where data is present, second is Client Org from where we callout to Server Org for data
Business Requirement: Establish connection between two Salesforce Developer orgs for data read and write
On First Org - Data Provider Org / Server Org
Step 1: Create Connected Apps on Source / Data Provider Org / Server Org
In order to allow external systems to access Salesforce, we need to provide them the authentication parameters.
We need to create a Connected App which will act as a gateway for external systems to access Salesforce data.
We will also get the Consumer key and Consumer Secret from the Connected App.
We need to make an http request using the Consumer Key and Consumer Secret to get the Bearer token.
Bearer token can be finally used to access Salesforce data by calling the Apex class AccountServiceAPI webservice we created.
This is how two step authentication OAuth works.
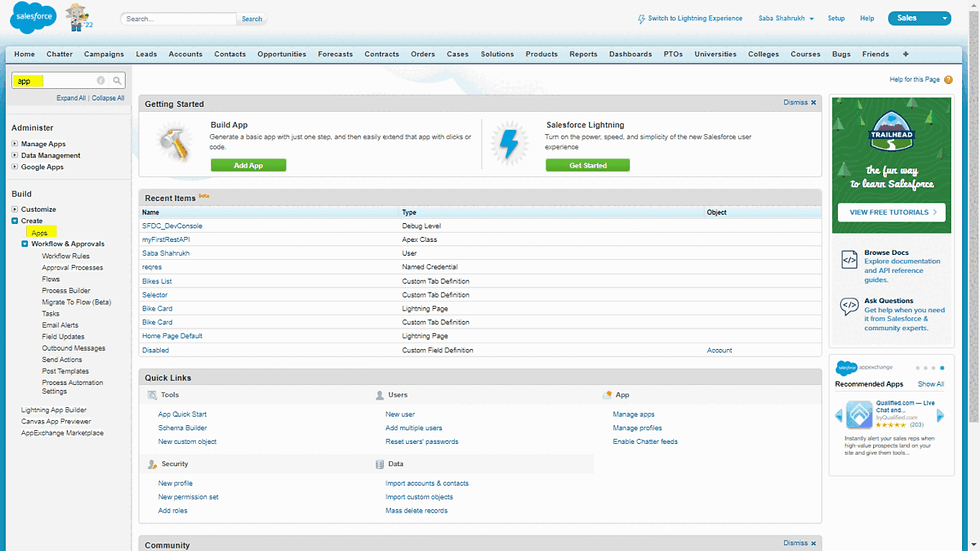
Step 2: Create a web service Apex Class in the Data Source / Data Provider Org / Server Org
//Any request to '/getAccountData/' will route to this class
//Make sure the class is global as it's method will be called from outside //this org
@RestResource(URLMapping='/getAccountData/*')
global class AccountDataManager{
@HttpGet //route the GET requests to this method
global static Account getAccount() {
//Get the RecordId from the Request URI > Fetch the record Data using
//SOQL > Return Data
RestRequest req = RestContext.request;
RestResponse res = RestContext.response;
String recordId =
req.requestURI.subString(req.requestURI.lastIndexOf('/')+1);
return [SELECT Id, Name FROM Lead WHERE Id=:recordId];
}
}
Step 3: Testing the API using Postman
URL to get initiate two step OAuth2 authentication to get the token
First we will test the webservice from Postman and then will create a REST API in another Salesforce org (Data Consumer org/ Client Org) to access the data from Data Provider org / Server Org.
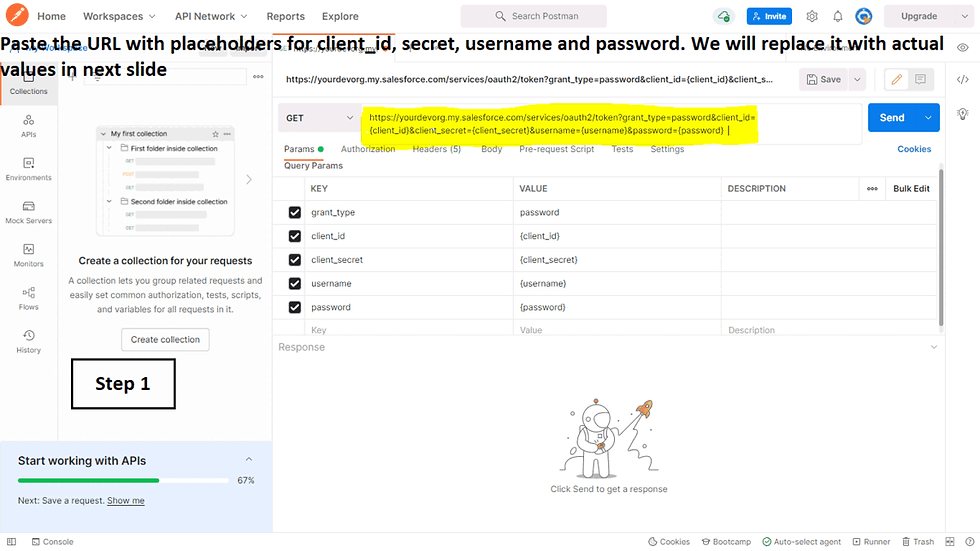
If you get data that means our Rest Resource is working fine, we are able to make Http request to our Data provider Salesforce org.
On Second Org - Data Consumer Org / Client Org
Now we need to make a Http Request from another Salesforce Org (Client Org) to access exposed data.
Step 1: Remote Site Setting on Data Consumer / Client Org
Now we need to inform Data Consumer Org / Client Org that we are going to make a callout from another Salesforce org (Data Provider Org) and we can do that by creating a Remote Site Setting with the endpoint URL of our Data Provider Org
Step 2: Rest API Callout from Apex class
We need to write a class to make exact same callout as we did from Postman
We need to make two callouts, first to get access token by passing Client Id and Client Secret in the Header
public class RestAPICallout{
public static void getAccountData(){
Http http = new Http();
HttpRequest req = new HttpRequest();
req.setMethod('POST');
//endpoint will contain client secret, client secret, username and password
req.setEndpoint('https://devOrg-dev-ed.my.salesforce.com/services/oauth2/token?grant_type=password&client_id={consumer_key_from_connected_app}&client_secret={consumer_secret_from_ connected_app}&username={org_username}&password={org_password}');
HttpResponse res = http.send(req);
//Parse the response and get the access_token
Oauth authResult = (Oauth) JSON.deserialize(res.getBody(), Oauth.class);
if(authResult.access_token != null){
Http newhttp = new Http();
HttpRequest request = new HttpRequest();
request.setMethod('GET');
//For now test by hard coded Account Id
request.setEndpoint('https://mydevorg-dev-ed.my.salesforce.com/services/apexrest/getAccountData/0016F*****Ftm');
request.setHeader('Content-type','application/JSON');
request.setHeader('Authorization','Bearer '+authResult.access_token);
HttpResponse response = newhttp.send(request);
//Check debug logs to see the response
System.debug(response.getBody());
}
}
public class Oauth{
public String access_token{get;set;}
public String instance_url{get;set;}
public String id{get;set;}
public String token_type{get;set;}
public String issued_at{get;set;}
public String signature{get;set;}
}
}
Step 3: Make callout
Open developer console and open Debug Anonymous Window and call RestAPICallout.getAccountData()
You can see the result in the Debug log.
API Connects is a global IT services firm in New Zealand brand excelling in Technology Architecture, Consulting, Software development & DevOps. Consult today! Visit: https://apiconnects.co.nz/devops-infrastructure-management/